Start developing
Introduction to building and styling apps with EDS.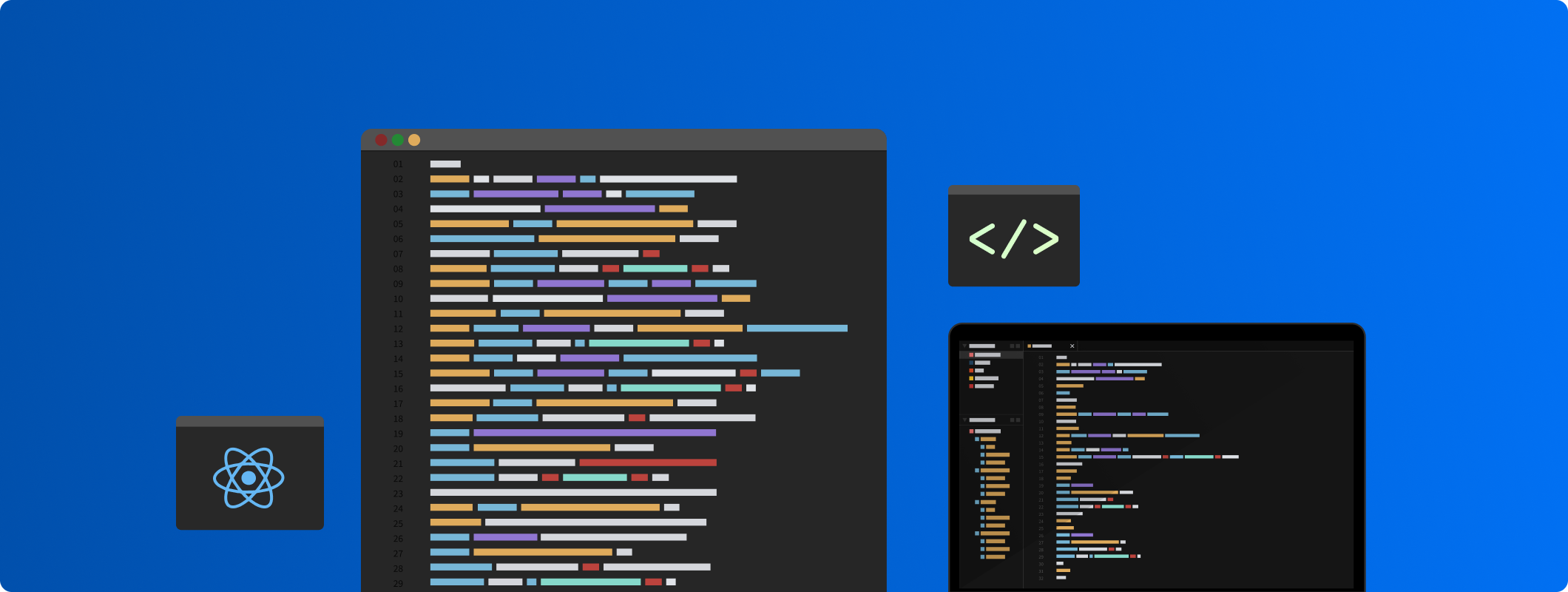
All EDS components are available as separate packages from Artifactory, and are prefixed with the @eds scope. To install our packages, ensure your application is set to install npm packages via Artifactory by running:
npm config set registry https://elmosoftware.jfrog.io/artifactory/api/npm/elmo-consolidated-npm/
EDS packages can now be installed using npm install @eds/THE-PACKAGE-NAME, e.g. npm install @eds/select-input. Package names can be found on their respective doc pages.
The @eds/core package provides the fundamentals to work with the design system, such as style tokens, theming and more. To start using EDS components in your app, you first need to install the Core component by running npm install @eds/core.
Once the package is installed, wrap your app in the <Core /> component high up in your component tree, for example where the app calls ReactDOM.render().
import { Core } from '@eds/core';ReactDOM.render(<Provider store={store}><Core><App /></Core></Provider>,document.getElementById('root'));
Read the Core documentation for more information on what the @eds/core package provides, how to use theme tokens and more.
In EDS, components are styled using a CSS-named prop system. Where applicable these "styling props" map to Tokens, which form the foundation of the EDS design language.
Take for example <Box backgroundColor="neutral" /> where;
- backgroundColor is the prop name – intuitively named after the CSS property, and
- neutral is the prop value - mapped to our semantically abstracted token.
Keep the Tokens page open in a tab as you develop, for quick and easy reference.
For styling props that doesn't map to any tokens, the native CSS property values are exposed as the prop values, for example the overflow prop accepts "auto" | "clip" | "hidden" | "scroll" | "visible" etc.
NOTE: Not all native CSS properties are available as styling props due to performance limits. Use the className prop in cases where you miss a certain styling prop.
The <Box /> component is our most primitive layout component, which supports all the styling props available in EDS. Most other components are built using the Box as a base.
Below is an example of how you can build a simple card component using the Box with styling props (for a full list see Box props).
const SimpleCard = () => { return ( <Box backgroundColor="neutral" borderColor="neutralSubtle" borderRadius="large" borderStyle="solid" borderWidth="thin" boxShadow="large" color="neutralSubtle" fontSize="medium" maxWidth="24rem" padding="large" > A simple card. </Box> ); }; return <SimpleCard />;
Other primitive layout components to check out are Flex and Grid, that both inherit all styling props from the Box. RailTrack is another useful layout component. It's encouraged to learn everything about these, since they will become your go-to tools for quickly composing EDS user interfaces.
Most styling props support the "responsive breakpoint syntax", which you can read more about on Responsive layouts. Try resizing your viewport width to see the backgroundColor change in the contrived example below.
<Box backgroundColor={{ small: 'positiveSubtle', large: 'informativeSubtle', xlarge: 'criticalSubtle', }} height="3rem" width="100%" />
You can also use our tokens directly in our files when you need to. They are all available to import from the @eds/core package, and can be implemented in conjunction with the className prop.
import { backgroundColor, color, space } from '@eds/core';
In cases where you need to define styles for CSS properties, pseudo-classes or pseudo-elements that aren't represented with a styling prop, you can reach for the className prop API that many of our components support.
EDS uses Emotion under the hood to compile all our styles into class names with CSS. Simply pass the css() function from the @emotion/css package to the prop, with an object of your styles as the argument.
Whilst this gives you a lot of freedom, it's encouraged that you still import and use our tokens as much as possible. In the example below, the background color is switched to backgroundColor.neutralInverted on :hover using the className prop.
// import { css } from '@emotion/css'; // import { backgroundColor } from '@eds/core'; const HoverCard = () => { return ( <Box backgroundColor="neutral" borderColor="neutralSubtle" borderRadius="large" borderStyle="solid" borderWidth="thin" boxShadow="large" color="neutralSubtle" fontSize="medium" maxWidth="24rem" padding="large" className={css({ '&:hover': { backgroundColor: backgroundColor.neutralInverted, }, })} > A card with className :hover styles. </Box> ); }; return <HoverCard />;
NOTE: Sometimes you need to type-cast your styles object, which you can easily do with the CSSType available from the @eds/core package.
const positionStyleExample: CSSType = {position: 'absolute',};
EDS does not support IE11 as it's no longer supported by Microsoft and is in the process of being phased out at ELMO. If you have any questions about browser support post a message in the Frontend Guild Slack channel.
If you have any questions or feedback about developing with EDS, you can contact the team through the links below. We encourage keeping conversations in the open, and including the whole team in discussions as much as possible.